Web Parsing Mistakes: Expert Guide to Error-Free Data Extraction (2025)
Coronium Technical Team
Web Scraping & Proxy Specialists
Web parsing (also known as web scraping) has become an essential tool for businesses seeking to extract valuable data from the internet. However, without proper implementation, your parsing projects can quickly run into roadblocks. Our technical team, with over 5 years of experience in proxy infrastructure and data extraction, has compiled this comprehensive guide to help you avoid the most common parsing mistakes and optimize your data collection operations.
What You'll Learn
- How to respect website rules & avoid legal issues
- Proper IP rotation strategies using mobile proxies
- Advanced CAPTCHA handling techniques
- Efficient dynamic content processing
- Optimized data storage architectures
- Setting natural request intervals
- Robust error handling strategies
- Future-proof web parsing architecture
Introduction to Web Parsing Challenges
Web parsing has evolved significantly in recent years as websites implement increasingly sophisticated anti-bot measures. What once required simple HTTP requests now demands advanced technologies and strategic approaches. Our team has observed that even experienced developers frequently encounter the same critical mistakes that compromise their data extraction efforts.
In this comprehensive guide, we'll explore the eight most common web parsing mistakes and provide actionable solutions based on our extensive experience with large-scale data extraction projects across various industries. By following these expert recommendations, you'll be able to build more reliable, efficient, and ethical web parsing systems.
Mistake #1: Ignoring Website Terms and Robots.txt
Disregarding a website's rules is not only ethically questionable but can lead to legal consequences and permanent IP bans.
Many developers jump straight into parsing without checking the website's robots.txt file or terms of service. This oversight can lead to legal issues, IP bans, and reputation damage. Websites invest heavily in their content and have legitimate reasons to protect it.
The Solution:
- Always check the robots.txt file - Before starting any parsing project, examine the website's robots.txt file to understand which areas are off-limits.
- Review terms of service - Many websites explicitly mention data scraping in their terms. Take time to understand these conditions.
- Consider API alternatives - Many sites offer official APIs that provide structured data access without violating terms.
- Respect rate limits - If mentioned in robots.txt or terms, adhere strictly to the specified request limits.
Here's a simple example of how to check a website's robots.txt file programmatically in Python before proceeding with parsing:
import requests
from urllib.robotparser import RobotFileParser
def is_crawling_allowed(url, user_agent="*"):
parsed_url = urlparse(url)
base_url = f"{parsed_url.scheme}://{parsed_url.netloc}"
# Initialize the RobotFileParser
rp = RobotFileParser()
rp.set_url(f"{base_url}/robots.txt")
try:
rp.read()
return rp.can_fetch(user_agent, url)
except Exception as e:
print(f"Error checking robots.txt: {e}")
# If there's an error, better to assume not allowed
return False
# Example usage
target_url = "https://example.com/data/page1"
if is_crawling_allowed(target_url):
# Proceed with scraping
print("Scraping is allowed!")
else:
print("Scraping is not allowed or could not determine permission")
Mistake #2: Using a Single IP Address
Relying on a single IP address for extensive parsing operations virtually guarantees you'll be blocked, often within minutes on security-conscious websites.
Modern websites can easily detect abnormal traffic patterns from a single IP address. Sending hundreds or thousands of requests from the same IP in a short period is a clear indicator of automated activity, which triggers protection systems.
The Solution:
- Implement IP rotation - Use a pool of proxies to distribute your requests across multiple IP addresses.
- Choose the right proxy type - Mobile proxies, like Coronium's 4G/5G solutions, offer carrier-grade IPs that are virtually indistinguishable from regular users.
- Configure session persistence when needed - For processes requiring login sessions, maintain the same IP throughout related operations.
- Implement smart rotation algorithms - Rotate IPs based on response codes, not just at fixed intervals.
Expert Tip: Mobile Proxies Advantage
Our testing across various websites shows that mobile proxies have a 95-99% success rate compared to 40-60% for datacenter proxies. This is because mobile IPs are shared among thousands of legitimate users, making your requests blend in with natural traffic patterns.
Mistake #3: Poor CAPTCHA Handling
CAPTCHAs are designed to distinguish humans from bots, and they've become increasingly sophisticated. Many parsing projects fail because they don't have a strategy for handling these challenges.
The Solution:
- Use CAPTCHA solving services - Services like 2Captcha, Anti-Captcha, or CapMonster can automatically solve most common CAPTCHAs.
- Implement intelligent retry mechanisms - When a CAPTCHA is detected, develop a system to handle it appropriately rather than continuing to make failed requests.
- Reduce CAPTCHA triggers - Using high-quality mobile proxies and natural request patterns significantly reduces CAPTCHA occurrences.
- Consider hybrid approaches - For critical operations, implement a system where difficult CAPTCHAs can be passed to human operators.
Mistake #4: Inadequate Dynamic Content Handling
Modern websites heavily rely on JavaScript to load content dynamically, which means simple HTTP request libraries like Requests or Axios often can't access the full page content.
The Solution:
- Use headless browsers - Tools like Puppeteer, Playwright, or Selenium can render JavaScript just like a real browser.
- Identify API endpoints - Often, the content loaded dynamically comes from internal APIs that you can access directly.
- Implement smart waiting strategies - Wait for specific elements to appear rather than using fixed timeouts.
- Consider using specialized frameworks - Tools like Crawlee, Scrapy with Splash, or ScrapingBee handle much of the complexity for you.
// Example using Puppeteer for JavaScript-heavy sites
const puppeteer = require('puppeteer');
const proxyChain = require('proxy-chain');
async function scrapeWithProxies() {
// Use mobile proxy with authentication
const oldProxyUrl = 'http://username:password@proxy.coronium.io:9000';
const newProxyUrl = await proxyChain.anonymizeProxy(oldProxyUrl);
const browser = await puppeteer.launch({
headless: true,
args: [
`--proxy-server=${newProxyUrl}`,
'--no-sandbox',
'--disable-setuid-sandbox'
]
});
try {
const page = await browser.newPage();
// Set realistic user agent
await page.setUserAgent('Mozilla/5.0 (iPhone; CPU iPhone OS 14_6 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/14.0.3 Mobile/15E148 Safari/604.1');
// Wait for network to be idle to ensure dynamic content loads
await page.goto('https://example.com', {
waitUntil: 'networkidle2',
timeout: 30000
});
// Wait for specific element that indicates content is loaded
await page.waitForSelector('.content-loaded', { timeout: 15000 });
// Extract data
const data = await page.evaluate(() => {
const items = Array.from(document.querySelectorAll('.item'));
return items.map(item => {
return {
title: item.querySelector('.title')?.textContent.trim(),
price: item.querySelector('.price')?.textContent.trim(),
// Extract more fields as needed
};
});
});
return data;
} finally {
await browser.close();
await proxyChain.closeAnonymizedProxy(newProxyUrl, true);
}
}
Mistake #5: Inefficient Data Storage Strategy
Many parsing projects collect data without a clear strategy for storing and processing it efficiently, leading to duplicate data, inconsistent formats, and difficulties in analysis.
The Solution:
- Develop a clear data schema - Before starting, define exactly what data you need and how it should be structured.
- Choose appropriate storage formats - Consider CSV for simple data, JSON for nested structures, or databases for complex relationships.
- Implement incremental processing - Process and store data as you go rather than keeping everything in memory.
- Maintain data provenance - Always store metadata about when and where the data was collected.
- Consider data validation - Implement checks to ensure the parsed data meets your expectations before storage.
Mistake #6: Unnatural Request Patterns
Making requests at perfectly timed intervals is a clear indicator of automated activity. Modern protection systems easily detect these patterns.
Bots typically make requests at perfectly regular intervals, while human browsing patterns show natural variation. Advanced protection systems can easily identify these mechanical patterns and flag your activity as automated.
The Solution:
- Implement variable delays - Use randomized intervals between requests that mimic human browsing patterns.
- Consider time-of-day variations - Adjust your parsing activity based on typical usage patterns for the target website.
- Simulate realistic user flows - Don't just request target pages; navigate through the site like a real user would.
- Implement exponential backoff - When encountering errors or rate limits, increase delay times progressively.
import random
import time
from typing import List, Optional
def human_like_delay() -> float:
"""Generates a random delay between 2-7 seconds with natural distribution."""
# Base delay between 2-4 seconds
base_delay = 2 + random.random() * 2
# Add random spike delay (20% chance of longer pause)
if random.random() < 0.2:
return base_delay + random.random() * 3
return base_delay
def request_with_backoff(url: str, max_retries: int = 3, proxies: Optional[List[str]] = None):
"""Make request with exponential backoff retry strategy and proxy rotation."""
retry_count = 0
delay = 1 # Initial delay in seconds
while retry_count < max_retries:
try:
# Rotate proxies if available
current_proxy = random.choice(proxies) if proxies else None
# Make the request with current proxy
response = requests.get(
url,
proxies={'http': current_proxy, 'https': current_proxy} if current_proxy else None,
timeout=10
)
# Check for success
if response.status_code == 200:
return response
# Handle specific error codes
if response.status_code == 429: # Too Many Requests
print(f"Rate limited, backing off...")
elif response.status_code in (403, 503): # Possible anti-bot measures
print(f"Possible bot detection, changing approach...")
except Exception as e:
print(f"Request error: {e}")
# Calculate backoff delay with randomization
backoff_delay = delay * (1 + random.random())
print(f"Retrying in {backoff_delay:.2f} seconds...")
time.sleep(backoff_delay)
# Increase delay for next potential retry (exponential backoff)
delay *= 2
retry_count += 1
raise Exception(f"Failed to get response after {max_retries} retries")
Mistake #7: Inadequate Error Handling
Web parsing operations encounter various errors - network issues, site changes, captchas, and more. Without robust error handling, your parser will frequently break down completely.
The Solution:
- Implement comprehensive try-except blocks - Catch and handle specific exceptions rather than using generic handlers.
- Develop intelligent retry logic - Some errors (like network timeouts) should trigger retries, while others (like 404 errors) should not.
- Log error details - Maintain detailed logs to help diagnose and fix issues.
- Implement circuit breakers - If a particular pattern of errors emerges, pause operations to prevent IP bans or resource wastage.
- Develop fallback strategies - When one approach fails, try alternative methods to get the needed data.
Mistake #8: Neglecting Maintenance and Adaptation
Websites constantly evolve, changing their structure, adding new protection measures, and updating their content. A parser that works perfectly today may completely fail tomorrow.
The Solution:
- Implement continuous monitoring - Set up automated checks to verify your parser is still functioning correctly.
- Design for flexibility - Build your parser with modular components that can be updated independently.
- Use robust selectors - Rely on stable identifiers rather than position-based or CSS class-based selectors that might change.
- Schedule regular reviews - Periodically examine your parsing infrastructure to identify potential improvements or vulnerabilities.
Advanced Web Parsing Strategies for 2025
Fingerprint Management
Beyond IP addresses, modern anti-bot systems analyze browser fingerprints. Manage canvas fingerprints, WebRTC, font detection, and other tracking vectors to remain undetected.
- Use tools like Puppeteer-extra-plugin-stealth
- Randomize browser dimensions and time zones
- Maintain consistent fingerprints per session
Headless Detection Evasion
Websites increasingly check for headless browser indicators. Modify your setup to pass these checks and appear as a regular browser.
- Override the navigator.webdriver property
- Emulate user interactions like mouse movements
- Use undetected-chromedriver for Selenium
Distributed Architecture
Scale your parsing operations with distributed systems that spread the load across multiple servers and IP ranges.
- Use message queues like RabbitMQ or Kafka
- Implement worker pools with autoscaling
- Centralize proxy and session management
Machine Learning Integration
Apply AI to both improve data extraction and evade detection by mimicking human behavior patterns.
- Use ML for unstructured data extraction
- Model human browsing patterns
- Implement adaptive rate limiting based on site response
Mobile Proxies: The Ultimate Web Parsing Solution
Our extensive testing has shown that mobile proxies provide the highest success rates for web parsing operations. Unlike datacenter IPs which are easily detected, mobile proxies use real carrier IPs that blend seamlessly with genuine user traffic.
- 95-99% success rate compared to 40-60% with datacenter proxies
- Authentic carrier IPs from providers like Verizon, AT&T, and T-Mobile
- Precise location targeting with city-level accuracy
- Significantly fewer CAPTCHAs and almost no IP blocks
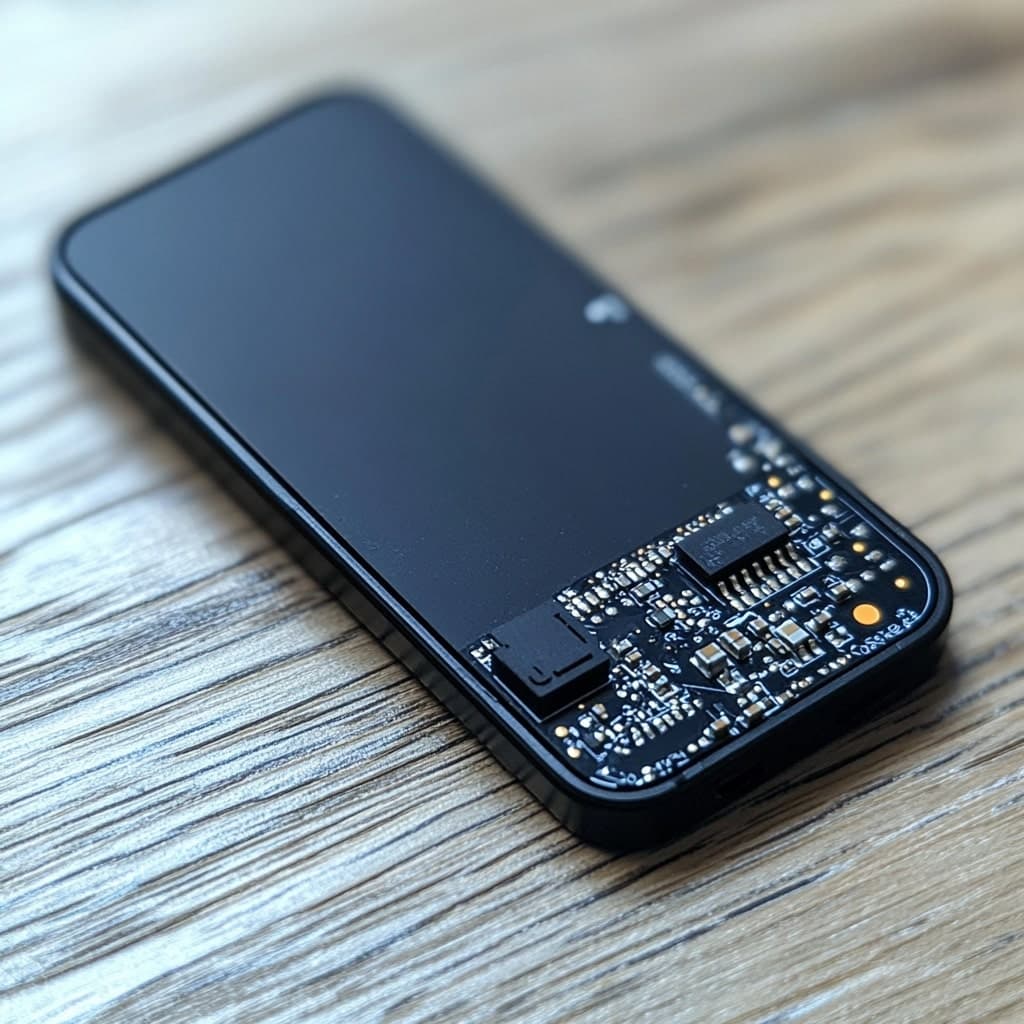
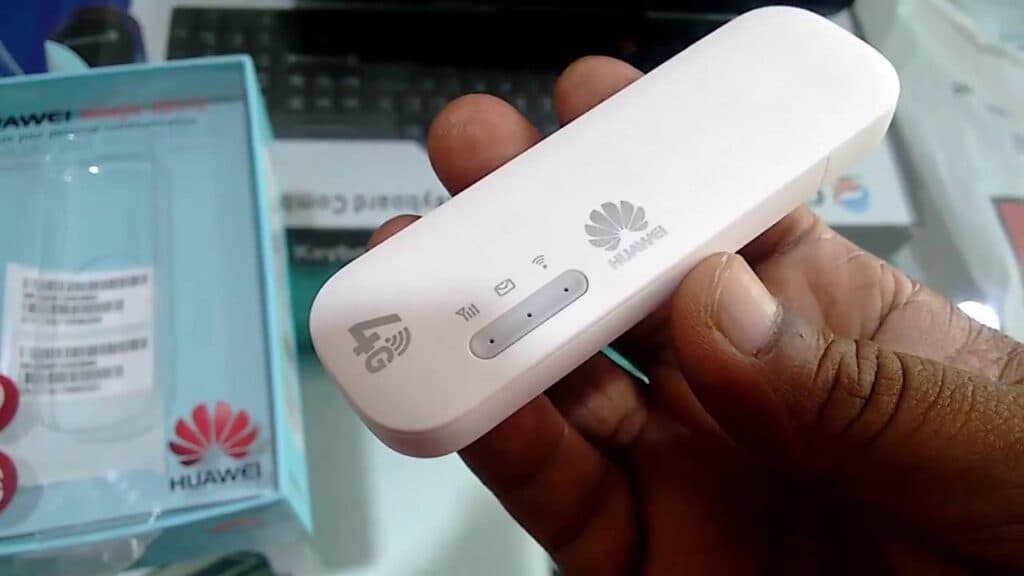
Frequently Asked Questions
What are the most common mistakes in web parsing?
The most common web parsing mistakes include ignoring website terms of service and robots.txt directives, using a single IP address without rotation, improper handling of dynamic JavaScript content, inefficient data storage strategies, inadequate error handling, and making requests at unnatural intervals that trigger anti-bot systems.
How can I make my web parser more reliable?
To make your web parser more reliable, implement robust error handling with retry mechanisms, use proper proxy rotation with mobile or residential IPs, handle dynamic content with headless browsers, respect website robots.txt and terms of service, maintain natural request patterns, implement proper data verification, and use appropriate technologies like Selenium, Puppeteer, or Playwright for JavaScript-heavy sites.
What proxy solution works best for web parsing?
Mobile proxies with 4G/5G connections typically work best for web parsing as they provide authentic carrier IP addresses with high trust scores, making your requests appear as genuine mobile users. These proxies offer superior undetectability compared to datacenter IPs, significantly reduce CAPTCHA triggers and IP blocks, and provide better geographical targeting for location-specific data collection.
Is web parsing legal?
Web parsing legality exists in a gray area that depends on several factors: the website's terms of service, how you use the collected data, and your jurisdiction. Always check the robots.txt file and terms of service before parsing, consider using official APIs when available, and consult legal advice for commercial applications. Never use parsed data for illegal activities or to reproduce copyrighted content without permission.
What frameworks are best for parsing in 2025?
For 2025, the most advanced parsing frameworks include Crawlee (JavaScript), which offers intelligent request handling and browser integration; Scrapy with Playwright (Python), which combines Scrapy's powerful architecture with Playwright's modern browser automation; ScrapingBee and BrightData for serverless solutions; and custom solutions built with Puppeteer or Selenium for maximum flexibility. The best choice depends on your specific requirements, programming language preference, and scaling needs.
Related Articles

AI-Powered Web Data Collection: Advanced Guide for 2025
Learn how AI is revolutionizing web data collection with intelligent extraction, processing, and analysis techniques that maximize efficiency while minimizing detection.

Advanced Web Parsing Tools: The Ultimate Guide for 2025
Discover the most efficient and powerful web parsing tools of 2025. This comprehensive guide covers next-generation scrapers, AI integration, and expert strategies.

Parsing with Crawlee: Ultimate Guide to Web Scraping in 2025
Master web scraping with Crawlee - the comprehensive library for data extraction. Learn to handle dynamic sites, bypass CAPTCHAs, and use 4G mobile proxies.
Conclusion: Building Sustainable Web Parsing Systems
Web parsing remains an essential tool for businesses seeking to extract valuable data for analysis, competitive intelligence, and decision-making. By avoiding the common mistakes outlined in this guide and implementing our recommended solutions, you can develop robust, efficient, and ethical web parsing systems that deliver reliable results even as websites evolve their protection mechanisms.
Remember that web parsing is not just about technical implementation but also about respecting the ecosystem of the web. By following ethical practices, you ensure the sustainability of your operations and contribute to a healthier internet environment for everyone.
Our team at Coronium is dedicated to providing not only the best proxy solutions for web parsing but also the expertise and guidance to help you succeed in your data extraction projects. Whether you're just starting or looking to optimize an existing setup, we're here to support your journey.
Ready to Optimize Your Web Parsing?
Our mobile proxy solutions provide the highest success rates for data extraction with authentic carrier-grade IPs across 30+ countries. Whether you're building a new parser or optimizing an existing one, our expert team can help.
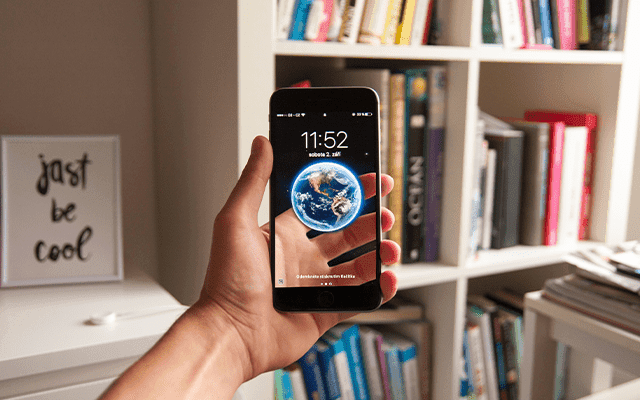
Mobile Proxies: Authentic Carrier IPs