ScapeGraph AI: Intelligent Web Scraping with LLMs
Introduction
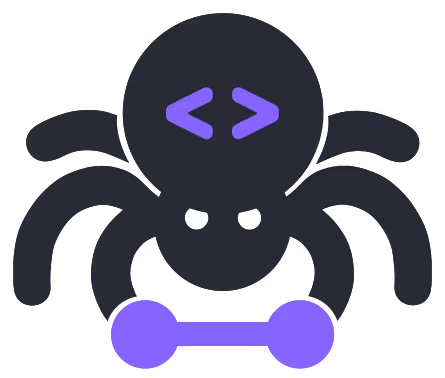
The web scraping landscape has been revolutionized by the integration of artificial intelligence. Traditional web scraping methods often rely on rigid selectors and patterns that break when websites change their structure. This creates a maintenance burden for developers who must constantly update their scrapers to adapt to these changes.
ScapeGraph AI represents a paradigm shift in this field by leveraging the power of Large Language Models (LLMs) to create intelligent, flexible web scraping pipelines. Rather than depending on fixed patterns, ScapeGraph AI understands the content it's extracting, making it resilient to changes in website layouts and structures.
What is ScapeGraph AI?
ScapeGraph AI is an open-source Python library designed to transform web scraping through the application of advanced AI techniques. Unlike traditional scraping tools that break when websites change their HTML structure, ScapeGraph AI uses the natural language understanding capabilities of Large Language Models to extract data based on meaning rather than fixed patterns.
The core concept is simple yet powerful: instead of writing complex selectors, you simply describe what information you want to extract in natural language. ScapeGraph AI then handles the technical details of finding and extracting that information, regardless of how it's structured in the source.
Key Benefits of ScapeGraph AI
- Adaptability: Automatically adjusts to changes in website structures and layouts
- Simplicity: Requires minimal configuration and coding compared to traditional scrapers
- Intelligence: Understands the context and meaning of the content being scraped
- Flexibility: Works with various document types including HTML, XML, JSON, and Markdown
- Extensibility: Supports multiple LLM providers and configurations
At its core, ScapeGraph AI implements a graph-based architecture where each node in the graph represents a specific operation in the scraping pipeline. This modular approach allows for incredible flexibility in how data is extracted, processed, and structured.
Key Features
AI-Powered Scraping
Uses LLMs to understand and extract data based on meaning rather than fixed patterns, making scrapers more resilient to website changes.
Graph-Based Architecture
Modular pipeline design allows complex scraping tasks to be broken down into manageable, interconnected operations.
Multiple LLM Support
Compatible with OpenAI, Azure OpenAI, Google Gemini, Anthropic Claude, Mistral AI, and local models via Ollama.
Multi-Format Support
Extracts data from HTML, XML, JSON, and Markdown files using the same intuitive interface.
Specialized Scrapers
Includes SmartScraperGraph, SearchGraph, and SpeechGraph for different extraction scenarios.
Developer-Friendly
Simple Python API with minimal configuration required to get started with advanced scraping capabilities.
These features combine to create a web scraping solution that's not only powerful but also adaptable to changing websites and requirements. The intelligent nature of ScapeGraph AI reduces the maintenance burden traditionally associated with web scrapers, allowing developers to focus on extracting value from the data rather than constantly updating their code.
How ScapeGraph AI Works
ScapeGraph AI's approach to web scraping is fundamentally different from traditional methods. Instead of relying on CSS selectors or XPath expressions that break when websites change, it uses Large Language Models to understand the content and extract the relevant information regardless of the specific HTML structure.
The Core Pipeline
- 1
Content Acquisition
The library fetches content from a website or loads it from a local file.
- 2
Content Processing
The content is preprocessed, chunked if necessary to handle token limits, and prepared for the LLM.
- 3
LLM Analysis
The LLM interprets the content and the user's extraction prompt to understand what data needs to be extracted.
- 4
Intelligent Extraction
The system extracts the specified information based on the LLM's understanding, not on fixed patterns.
- 5
Result Formatting
The extracted data is structured according to the user's requirements and returned as the final output.
Graph-Based Architecture
The name "ScapeGraph" comes from the library's graph-based pipeline architecture. Each stage in the scraping process is represented as a node in a directed graph, with edges representing the flow of data between stages. This approach offers several advantages:
- Modularity: Each node performs a specific function, allowing for easy customization and extension.
- Flexibility: Pipelines can be reconfigured by changing the connections between nodes.
- Reusability: Common operations can be encapsulated in reusable nodes.
- Transparency: The graph structure makes it clear how data flows through the system.
LLM Integration
ScapeGraph AI supports multiple LLM providers, allowing users to choose the model that best fits their needs and budget. The library handles the complexities of token limits, prompt engineering, and response parsing, making it easy to leverage the power of LLMs without getting bogged down in technical details.
Installation and Setup
Getting started with ScapeGraph AI is straightforward. The library requires Python and can be installed using pip.
Prerequisites
- Python 3.9 or higher
- Pip package manager
Installation Steps
ScapeGraph AI can be installed using pip, and if you need browser-based scraping capabilities, you'll also need to install Playwright. The installation process is straightforward and well-documented in the project's GitHub repository.
Configuration
After installation, you'll need to configure ScapeGraph AI to use your preferred LLM provider. The library supports various providers including OpenAI, Azure OpenAI, Google Gemini, Anthropic Claude, Mistral AI, and local models via Ollama, each with its own configuration requirements.
Other supported providers include Azure OpenAI, Google Gemini, Anthropic Claude, and Mistral AI, each with similar configuration patterns. The documentation provides detailed examples for each provider.
Basic Usage Examples
ScapeGraph AI provides an intuitive API that makes it easy to extract data from websites. The following examples demonstrate common usage patterns.
Basic Usage
ScapeGraph AI provides an intuitive API that makes it easy to extract data from websites. You can extract information from websites using the SmartScraperGraph, extract data from local files, and request structured data in specific formats like JSON.
The library allows you to specify what information you want to extract using natural language prompts, and it handles the technical details of finding and extracting that information from the source content.
Advanced Techniques
ScapeGraph AI offers advanced features for more complex scraping scenarios. These techniques allow you to handle larger websites, customize the scraping process, and integrate with other tools.
Advanced Capabilities
ScapeGraph AI offers advanced features for more complex scraping scenarios:
- Search-Based Extraction: The SearchGraph allows you to search for specific information within larger documents
- Speech Processing: SpeechGraph can process audio content and extract information from it
- Pagination Handling: For websites with paginated content, you can iteratively scrape multiple pages
- Custom Graph Pipelines: Advanced users can create custom graph pipelines for specialized scraping needs
These techniques allow you to handle larger websites, customize the scraping process, and integrate with other tools for a comprehensive data extraction workflow.
Using ScapeGraph AI with Proxies
When scraping websites at scale, you may encounter rate limits or IP blocks. Using proxies can help overcome these limitations by distributing your requests across multiple IP addresses. ScapeGraph AI can be configured to work with proxies, including mobile proxies from Coronium.io.
Why Use Proxies with ScapeGraph AI?
- Avoid Rate Limiting: Distribute requests across multiple IPs to avoid triggering rate limits.
- Bypass IP Blocks: Continue scraping even if some IPs are blocked by the target website.
- Geo-Targeting: Access location-specific content by using proxies from different regions.
- Enhanced Privacy: Hide your real IP address for more anonymous scraping.
Configuring Proxies in ScapeGraph AI
ScapeGraph AI supports proxy configuration through its underlying HTTP libraries. Here's how to set up proxies:
Using Mobile Proxies with ScapeGraph AI
Mobile proxies provide IP addresses from mobile carriers, which are less likely to be blocked by websites. Coronium.io offers reliable mobile proxies that work seamlessly with ScapeGraph AI. The library supports proxy configuration through its underlying HTTP libraries, allowing you to use proxies for enhanced scraping capabilities.
You can easily configure ScapeGraph AI to use mobile proxies from different regions, rotate between multiple proxies, and customize your scraping infrastructure to avoid detection and improve success rates.
Why Choose Coronium Mobile Proxies
Coronium.io's mobile proxies offer several advantages for AI-powered web scraping:
- High Success Rates: Mobile IPs are less likely to be blocked by websites, improving scraping reliability.
- Geographic Diversity: Access to IPs from multiple countries for geo-targeted scraping.
- Stable Connections: Reliable infrastructure ensures consistent performance for your ScapeGraph AI applications.
- Flexible Authentication: Simple username/password authentication that integrates easily with ScapeGraph AI.
- Rotating IPs: Options for both static and rotating IPs to suit different scraping strategies.
Use Cases for ScapeGraph AI
ScapeGraph AI's intelligent approach to web scraping opens up a wide range of applications across different industries. Here are some of the most common use cases:
E-commerce Monitoring
Track competitor prices, product availability, and promotions across multiple online stores to inform pricing and inventory decisions.
Market Research
Gather product reviews, ratings, and market trends from various sources to understand consumer sentiment and market dynamics.
Content Aggregation
Collect and organize content from multiple websites to create news aggregators, research databases, or specialized information portals.
Data Enrichment
Enhance existing datasets with additional information scraped from the web, providing more context and value for analysis.
Real-World Examples
Financial Analysis
A financial research firm uses ScapeGraph AI to extract earnings reports, analyst estimates, and financial news from various sources. The AI-powered approach allows them to quickly gather and process information even when websites change their layouts.
# Financial data extraction example
from scrapegraphai.graphs import SmartScraperGraph
scraper = SmartScraperGraph(
prompt="""
Extract the following financial metrics for Q3 2024:
1. Revenue
2. Net Income
3. EPS (Earnings Per Share)
4. Revenue Growth (YoY)
5. Profit Margin
Also extract any forward guidance for Q4 2024.
""",
source="https://example-financial-site.com/earnings/company-xyz",
config=graph_config
)
financial_data = scraper.run()
print(financial_data)
Real Estate Market Analysis
A real estate analytics company uses ScapeGraph AI to track property listings, prices, and features across multiple listing websites. This data helps identify market trends and provide insights to buyers and sellers.
# Real estate data extraction
from scrapegraphai.graphs import SmartScraperGraph
scraper = SmartScraperGraph(
prompt="""
Extract all property listings with the following details:
1. Property type (house, apartment, etc.)
2. Price
3. Location (address and neighborhood)
4. Square footage
5. Number of bedrooms and bathrooms
6. Year built
7. Special features or amenities
Format the data as a JSON array of objects.
""",
source="https://example-realestate.com/listings?city=newyork",
config=graph_config
)
property_listings = scraper.run()
print(property_listings)
Research Paper Analysis
Academic researchers use ScapeGraph AI to extract key information from research papers, including methodologies, findings, and citations. This helps them stay current with the latest developments in their field.
# Research paper analysis
from scrapegraphai.graphs import SearchGraph
search_graph = SearchGraph(
query="""
What are the main findings related to transformer architecture improvements
in NLP research papers published in the last two years?
Summarize the key innovations and performance improvements.
""",
source="https://example-academic-repository.com/papers/computer-science/nlp",
config=graph_config
)
research_findings = search_graph.run()
print(research_findings)
Conclusion
ScapeGraph AI represents a significant advancement in web scraping technology. By leveraging the power of Large Language Models and a flexible graph-based architecture, it offers a more intelligent, adaptable approach to data extraction that can handle changes in website structures without requiring constant maintenance.
The key advantages of ScapeGraph AI include:
- Reduced Maintenance: Less need to update scraping code when websites change their layout.
- Simplified Development: Natural language prompts instead of complex selectors and patterns.
- Flexibility: Support for multiple document types and LLM providers.
- Scalability: Ability to handle complex scraping tasks through custom graph pipelines.
When combined with reliable mobile proxies from Coronium.io, ScapeGraph AI becomes an even more powerful tool for web data extraction, offering improved success rates, geo-targeting capabilities, and enhanced privacy.
Enhance Your AI-Powered Web Scraping Today
Whether you're a data scientist, market researcher, or developer, combining ScapeGraph AI with Coronium's mobile proxies gives you a powerful toolkit for reliable and efficient web data extraction. Get started today to experience the benefits of intelligent, adaptive web scraping.
About the Author
Coronium.io Organization
Coronium.io is a leading provider of advanced networking solutions, specializing in proxy services and VPN technologies. Committed to innovation and user satisfaction, Coronium.io offers tools that enhance online privacy, security, and performance for individuals and businesses alike.
Disclaimer
Our 4G mobile proxies are intended for legal and legitimate use only. This page is solely for informational and marketing purposes. It is the user's responsibility to ensure compliance with the terms of service of the platforms they are using our proxies on. We do not condone or support the use of our proxies for illegal or unauthorized activities. By using our proxies, you agree to use them in accordance with all applicable laws and regulations. We will not be held liable for any misuse of our proxies. Please read our Terms of Service before using our services.